LLM and Hugging Face: Text Summarization
Text Summarization condenses longer text into concise summaries with few lines of code.
In this blog, we’ll walk you through how to build a text summarization feature using Hugging Face’s pre-trained models and integrate it into a React frontend.
Why Text Summarization ?
Text summarization is the process yof breaking down longer text into shorter, coherent versions while retaining the key information. It’s incredibly useful for:
- Quickly understanding long articles or documents.
- Saving time on research and reading.
- Enhancing productivity by focusing on the most important details.
However, one thing to keep in mind is that some LLMs have a maximum input length. For example, the facebook/bart-large-cnn
model we’ll use can handle up to 1024 tokens (approximately 700-800 words).
If your text exceeds this limit, you’ll need to split it into smaller chunks.
Setting up Backend
Continue with the previous backend setup and route the Hugging Face Inference API key by writing following code.
// create route for hugging face api
app.post("/api/summarize", async (req, res) => {
const { text } = req.body;
try {
const response = await hf.summarization({
model: 'facebook/bart-large-cnn',
inputs: text,
})
res.status(200).json(response)
} catch(err) {
res.status(500).json({
message: `Error in summarizing text at backend ${err}`,
})
}
})
output format:
output: {
summary_text: '..........'
}
Setup Frontend UI [ REACT ]
In Summarize.js
component, create textarea
field and call your backend API using axios
.
import React, { useState } from 'react'
import axios from 'axios'
const Summarize = () => {
const [text, setText] = useState('')
const [summary, setSummary] = useState('')
const handleSummarize = async (e) => {
e.preventDefault()
try {
const response = await axios.post('http://localhost:5000/api/summarize', { text } )
setSummary(response.data)
} catch(err) {
setSummary({message: err})
}
}
const handleClear = () => {
setSummary('')
setText('')
}
return (
<>
<form onSubmit={handleSummarize}>
<h2> Summarize Text</h2>
<textarea
rows='10'
cols='50'
value={text}
onChange={(e) => setText(e.target.value)}
>
</textarea>
<div className='btns'>
<button>Summarize</button>
<button className='clear' onClick={handleClear}>Clear</button>
</div>
</form>
<div className='result'>
<h3>Summary</h3>
<p className='warning'>{summary.message && summary.message}</p>
<p className='result'>{ summary.summary_text }</p>
</div>
</>
)
}
export default Summarize
You can test the API tool using tools like Postman or cURL by sending a POST request to http://localhost:5000/api/summarize
with a JSON payload.
Run the application
- start the backend server:
node server.js
- start React app:
npm start
- Open browser and navigate to
http://localhost:3000
- Enter long text (e.g., Wikipedia paragraph about Earth) and click "Summarize." You’ll see the summarized text displayed below.
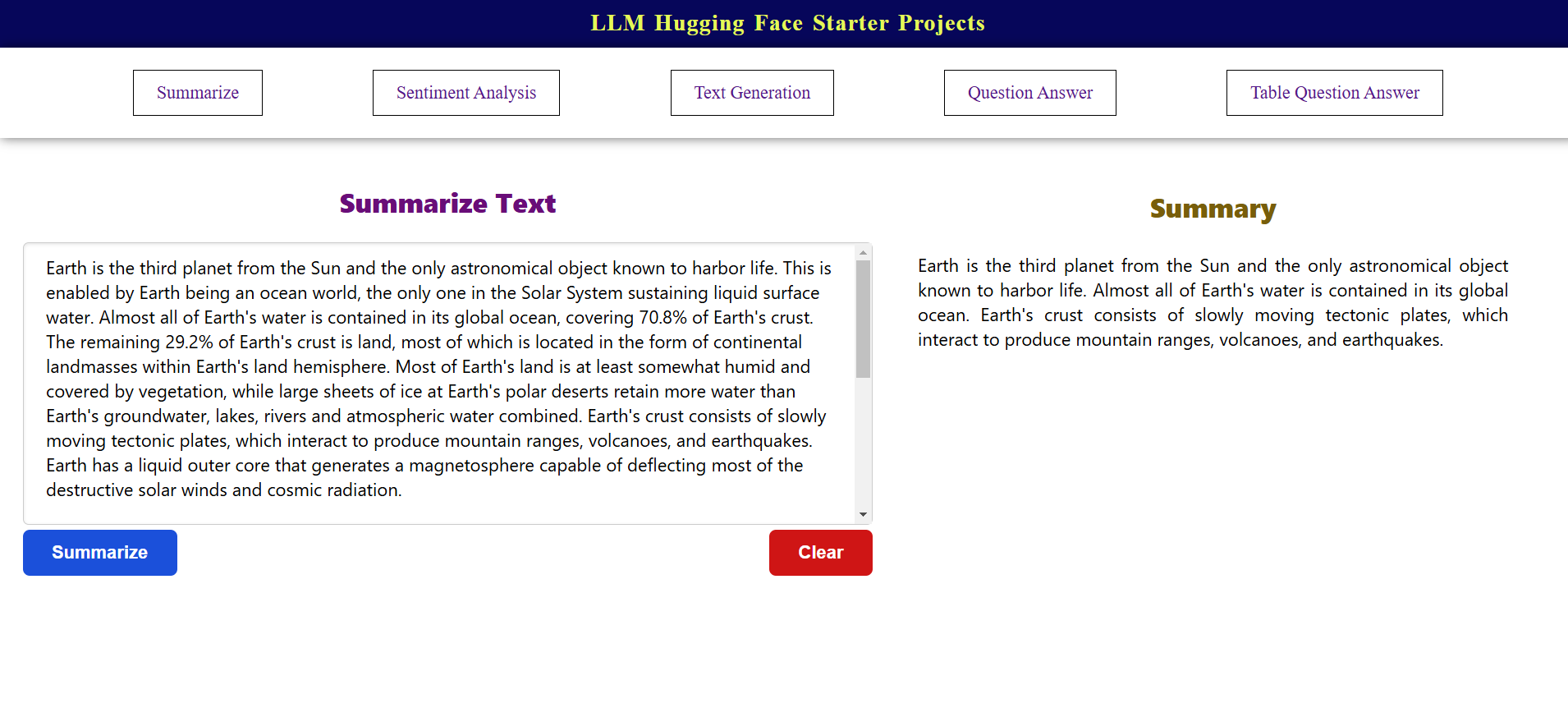